The basics of ArcPy#
ArcPy is a Python site package that provides a useful and productive way to perform:
geographic data analysis,
data conversion,
data management,and
map automation.
In ArcGIS Pro, most geoprocessing functions can be accessed through ArcPy.
Similar to ModelBuilder which offers a visually straightforward way to automate complex process, we can connect multiple ArcPy functions and customize the workflow as an alternative way to automate geoprocessing.
1. ArcPy Workspace#
Similar to the random
or sys
module that we have used before, arcpy
package
has to be imported, i.e., import arcpy
, before we can access its functions and methods.
import arcpy
1.1 What is a workspace#
In ArcGIS, a workspace is a container for geographic data. A workspace can be a folder that contains shapefiles, a geodatabase, a feature dataset, or an ArcInfo workspace.
To reference a workspace, one needs to specify the path to the directory where the workspace is located.
Methods to quickly grab a path
In File Explorer: right click
a folder then Copy as path, or Ctrl + Shift + C
.
In ArcGIS Pro: Map Tab -> Clipboard -> Copy Path, or Ctrl + Alt + P
, this only
works when a workspace or dataset is selected in the “Catalog Pane.”
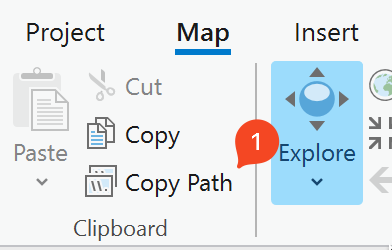
1.2 Working with paths#
The above methods copy the path with a backslash, i.e., \
as the delimiter
between a parent folder and a sub-folder, which is in conflict with the
escape character of Python.
There are three options to address this:
Change the delimiter to be double backslashes, i.e.,
\\
Change the delimiter to be a single forwardslash, i.e.,
/
Change the string to a raw string with a
r
in front of the path.
The following three strings are referring to the same path.
print("C:\\Users\\chjch\\Documents\\class_data.gdb") # double backslashes
print("C/Users/chjch/Documents/class_data.gdb") # single forward slash
print(r"C\Users\chjch\Documents\class_data.gdb") # raw string
C:\Users\chjch\Documents\class_data.gdb
C/Users/chjch/Documents/class_data.gdb
C\Users\chjch\Documents\class_data.gdb
# the following code will raise an SyntaxError
print('C\Users\chjch\Documents\class_data.gdb')
Cell In[3], line 3
print('C\Users\chjch\Documents\class_data.gdb')
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 1-2: truncated \UXXXXXXXX escape
Warning
Path names with spaces in them must be called with an ‘r’ and double quotes.
Note
Some other escape sequence in Python
\\
–> backslash ()\"
–> double quote\'
–> single quote\n
–> line feed (start a new line)\t
–> a Tab (eight spaces by default in Python)
print('hello\nworld')
print('\thello')
print(' world') # eight white spaces
hello
world
hello
world
1.3 Set workspace
of the env
object#
gdb_worksp = r"..\data\class_data.gdb"
arcpy.env.workspace = gdb_worksp
`workspace` is an **attribute** of the `env` class in the `arcpy` package
getattr(arcpy.env, "workspace")
'..\\data\\class_data.gdb'
type(arcpy.env)
arcpy.geoprocessing._base.GPEnvironments.<locals>.GPEnvironment
Use the dir
method to access all objects and methods with the env
class.
# view all attributes of the env class of ArcPy
print(dir(arcpy.env))
['MDomain', 'MResolution', 'MTolerance', 'S100FeatureCatalogueFile', 'XYDomain', 'XYResolution', 'XYTolerance', 'ZDomain', 'ZResolution', 'ZTolerance', '__class__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getstate__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__iter__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__setitem__', '__sizeof__', '__slots__', '__str__', '__subclasshook__', '_environments', '_gp', '_refresh', 'addOutputsToMap', 'annotationTextStringFieldLength', 'autoCancelling', 'autoCommit', 'baDataSource', 'baNetworkSource', 'baUseDetailedAggregation', 'buildStatsAndRATForTempRaster', 'cartographicCoordinateSystem', 'cartographicPartitions', 'cellAlignment', 'cellSize', 'cellSizeProjectionMethod', 'coincidentPoints', 'compression', 'configKeyword', 'daylightSaving', 'extent', 'geographicTransformations', 'gpuId', 'isCancelled', 'items', 'iteritems', 'keys', 'maintainAttachments', 'maintainCurveSegments', 'maintainSpatialIndex', 'mask', 'matchMultidimensionalVariable', 'nodata', 'outputCoordinateSystem', 'outputMFlag', 'outputZFlag', 'outputZValue', 'overwriteOutput', 'packageWorkspace', 'parallelProcessingFactor', 'preserveGlobalIds', 'processingServer', 'processingServerPassword', 'processingServerUser', 'processorType', 'pyramid', 'qualifiedFieldNames', 'randomGenerator', 'rasterStatistics', 'recycleProcessingWorkers', 'referenceScale', 'resamplingMethod', 'retryOnFailures', 'scratchFolder', 'scratchGDB', 'scratchWorkspace', 'scriptWorkspace', 'snapRaster', 'terrainMemoryUsage', 'tileSize', 'timeZone', 'tinSaveVersion', 'transferDomains', 'transferGDBAttributeProperties', 'unionDimension', 'useCompatibleFieldTypes', 'values', 'workspace']
2. Basic Things to Know about ArcPy#
2.1 Naming Conventions of ArcPy functions#
In ArcPy, a function is referenced by the geoprocessing (GP) tool name and the alias of the toolbox that the tool is contained. The first letter of every word in the tool name is capitalized. The alias of the toolbox are all in lowercase.
arcpy.<ToolName>_<toolboxalias>
(old way)Buffer:
arcpy.AddField_management
Add Field:
arcpy.AddField_management
arcpy.<toolboxalias>.<ToolName>
(modern way)Buffer:
arcpy.analysis.Buffer
Add Field:
arcpy.management.AddField
Following are the aliases for some commonly used toolbox.
System Toolbox |
Alias |
---|---|
Analysis |
|
Conversion |
|
Data Management |
|
Editing |
|
Geostatistical Analyst |
|
Network Analyst |
|
Spatial Analyst |
|
Spatial Statistics |
|
2.2 View Help Documentation#
There are 4 methods to view the Help Documentation of a function.
Shift + Tab
: open a popup menu in place.use the function’s
.__doc__
attributehelp(<function>)
? <function>
arcpy.analysis.Buffer()
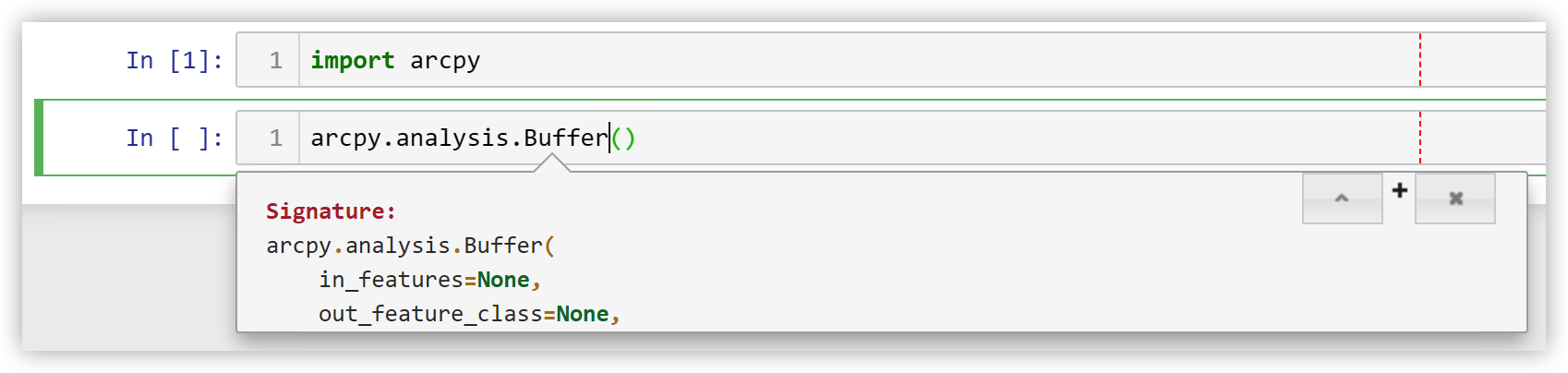
Note
To bring documentation as you type, press Shift + Tab
right after the
function name. It only works after the module or package containing the function is imported.
print(arcpy.Buffer_analysis.__doc__)
Buffer_analysis(in_features, out_feature_class, buffer_distance_or_field, {line_side}, {line_end_type}, {dissolve_option}, {dissolve_field;dissolve_field...}, {method})
Creates buffer polygons around input features to a specified distance.
INPUTS:
in_features (Feature Layer):
The input point, line, or polygon features that will be buffered.
buffer_distance_or_field (Linear Unit / Field):
The distance around the input features that will be buffered.
Distances can be provided as either a value representing a linear
distance or a field from the input features that contains the distance
to buffer each feature.If linear units are not specified or are
entered as Unknown, the
linear unit of the input features' spatial reference will be used.When
specifying a distance, if the linear unit has two words, such as
Decimal Degrees, combine the two words into one (for example, 20
DecimalDegrees).
line_side {String}:
Specifies the sides of the input features that will be buffered. This
parameter is only supported for polygon and line features.FULL-For
lines, buffers will be generated on both sides of the line.
For polygons, buffers will be generated around the polygon and will
contain and overlap the area of the input features. This is the
default.LEFT-For lines, buffers will be generated on the topological
left of
the line. This option is not supported for polygon input
features.RIGHT-For lines, buffers will be generated on the topological
right of
the line. This option is not supported for polygon input
features.OUTSIDE_ONLY-For polygons, buffers will be generated outside
the input
polygon only (the area inside the input polygon will be erased from
the output buffer). This option is not supported for line input
features.This optional parameter is not available with a Desktop Basic
or
Desktop Standard license.
line_end_type {String}:
Specifies the shape of the buffer at the end of line input features.
This parameter is not valid for polygon input features.ROUND-The ends
of the buffer will be round, in the shape of a half
circle. This is the default.FLAT-The ends of the buffer will be flat
or squared and will end at
the endpoint of the input line feature.This optional parameter is not
available with a Desktop Basic or
Desktop Standard license.
dissolve_option {String}:
Specifies the type of dissolve that will be performed to remove buffer
overlap.NONE-An individual buffer for each feature will be maintained,
regardless of overlap. This is the default.ALL-All buffers will be
dissolved together into a single feature,
removing any overlap.LIST-Any buffers sharing attribute values in the
listed fields
(carried over from the input features) will be dissolved.
dissolve_field {Field}:
The list of fields from the input features on which the output buffers
will be dissolved. Any buffers sharing attribute values in the listed
fields (carried over from the input features) will be dissolved.
method {String}:
Specifies whether the planar or geodesic method will be used to create
the buffers. PLANAR-If the input features are in a projected
coordinate
system, Euclidean buffers will be created. If the input features are
in a geographic coordinate system and the buffer distance is in linear
units (meters, feet, and so forth, as opposed to angular units such as
degrees), geodesic buffers will be created. This is the default.
You can use the Output Coordinate System environment setting to
specify the coordinate system to use. For example, if the input
features are in a projected coordinate system, you can set the
environment to a geographic coordinate system to create geodesic
buffers.GEODESIC-All buffers will be created using a shape-preserving
geodesic
buffer method, regardless of the input coordinate system.
OUTPUTS:
out_feature_class (Feature Class):
The feature class containing the output buffers.
help(arcpy.Buffer_analysis)
Help on function Buffer in module arcpy.analysis:
Buffer(in_features=None, out_feature_class=None, buffer_distance_or_field=None, line_side: "Literal['FULL', 'LEFT', 'RIGHT', 'OUTSIDE_ONLY'] | None" = None, line_end_type: "Literal['ROUND', 'FLAT'] | None" = None, dissolve_option: "Literal['NONE', 'ALL', 'LIST'] | None" = None, dissolve_field=None, method: "Literal['GEODESIC', 'PLANAR'] | None" = None) -> 'Result1[str]'
Buffer_analysis(in_features, out_feature_class, buffer_distance_or_field, {line_side}, {line_end_type}, {dissolve_option}, {dissolve_field;dissolve_field...}, {method})
Creates buffer polygons around input features to a specified distance.
INPUTS:
in_features (Feature Layer):
The input point, line, or polygon features that will be buffered.
buffer_distance_or_field (Linear Unit / Field):
The distance around the input features that will be buffered.
Distances can be provided as either a value representing a linear
distance or a field from the input features that contains the distance
to buffer each feature.If linear units are not specified or are
entered as Unknown, the
linear unit of the input features' spatial reference will be used.When
specifying a distance, if the linear unit has two words, such as
Decimal Degrees, combine the two words into one (for example, 20
DecimalDegrees).
line_side {String}:
Specifies the sides of the input features that will be buffered. This
parameter is only supported for polygon and line features.FULL-For
lines, buffers will be generated on both sides of the line.
For polygons, buffers will be generated around the polygon and will
contain and overlap the area of the input features. This is the
default.LEFT-For lines, buffers will be generated on the topological
left of
the line. This option is not supported for polygon input
features.RIGHT-For lines, buffers will be generated on the topological
right of
the line. This option is not supported for polygon input
features.OUTSIDE_ONLY-For polygons, buffers will be generated outside
the input
polygon only (the area inside the input polygon will be erased from
the output buffer). This option is not supported for line input
features.This optional parameter is not available with a Desktop Basic
or
Desktop Standard license.
line_end_type {String}:
Specifies the shape of the buffer at the end of line input features.
This parameter is not valid for polygon input features.ROUND-The ends
of the buffer will be round, in the shape of a half
circle. This is the default.FLAT-The ends of the buffer will be flat
or squared and will end at
the endpoint of the input line feature.This optional parameter is not
available with a Desktop Basic or
Desktop Standard license.
dissolve_option {String}:
Specifies the type of dissolve that will be performed to remove buffer
overlap.NONE-An individual buffer for each feature will be maintained,
regardless of overlap. This is the default.ALL-All buffers will be
dissolved together into a single feature,
removing any overlap.LIST-Any buffers sharing attribute values in the
listed fields
(carried over from the input features) will be dissolved.
dissolve_field {Field}:
The list of fields from the input features on which the output buffers
will be dissolved. Any buffers sharing attribute values in the listed
fields (carried over from the input features) will be dissolved.
method {String}:
Specifies whether the planar or geodesic method will be used to create
the buffers. PLANAR-If the input features are in a projected
coordinate
system, Euclidean buffers will be created. If the input features are
in a geographic coordinate system and the buffer distance is in linear
units (meters, feet, and so forth, as opposed to angular units such as
degrees), geodesic buffers will be created. This is the default.
You can use the Output Coordinate System environment setting to
specify the coordinate system to use. For example, if the input
features are in a projected coordinate system, you can set the
environment to a geographic coordinate system to create geodesic
buffers.GEODESIC-All buffers will be created using a shape-preserving
geodesic
buffer method, regardless of the input coordinate system.
OUTPUTS:
out_feature_class (Feature Class):
The feature class containing the output buffers.
? arcpy.analysis.Buffer